· 7 min read
Exponents in Python: A Quick Guide
In this blog, we will explore the importance of exponents in Python and different methods to calculate them using Python. We will compare each method's efficiency.
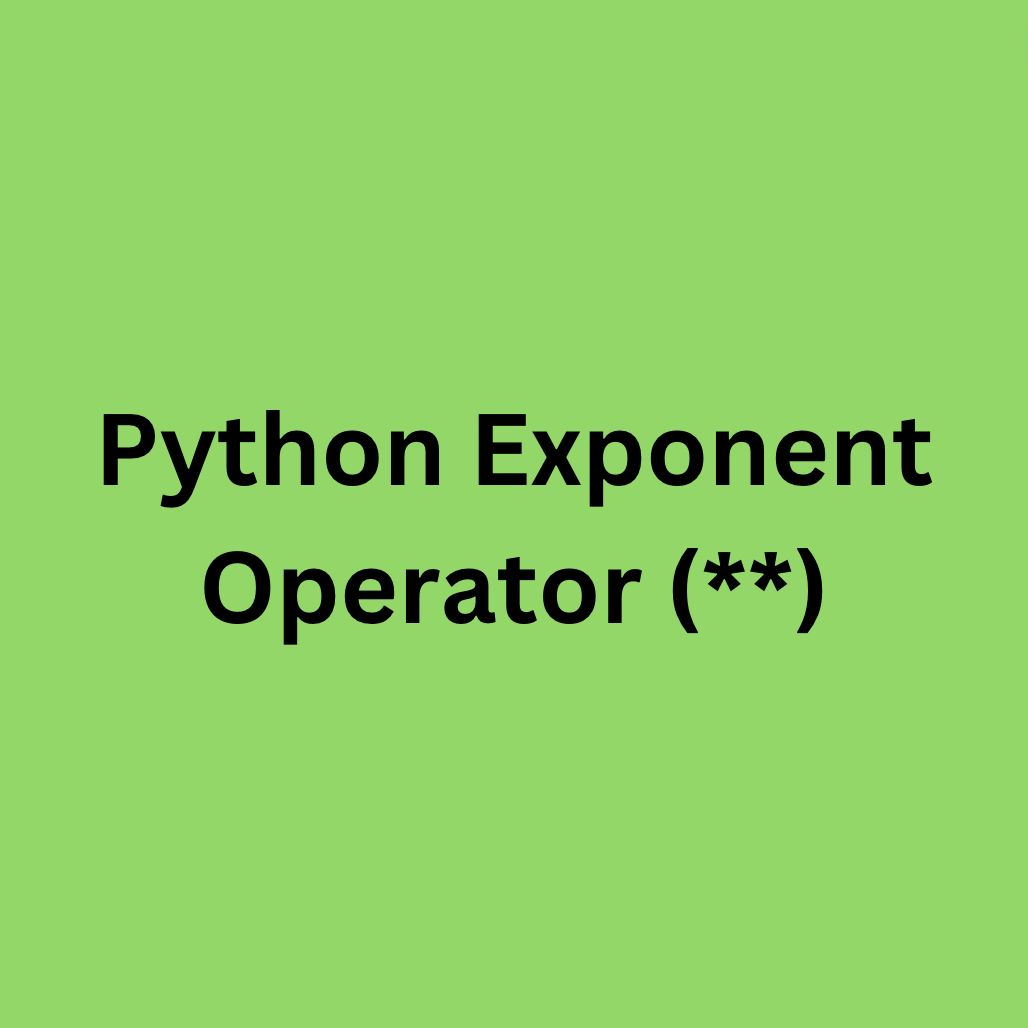
Exponents, which represent repeated multiplication, play a crucial role in performing complex calculations in Python.
In this blog, we will explore the importance of exponents and different methods to calculate them. We will compare each method’s efficiency and discuss common errors that programmers usually encounter while calculating exponents. We will also touch upon advanced techniques such as using complex numbers with exponents in Python.
What is an exponent in Python?
In Python, an exponent is a mathematical operator that raises a number to a certain power. It is represented by double-asterisk notation (**) and can be used to perform calculations such as squaring a number or raising it to any desired power.
Exponents in Python in real world
Exponents simplify complex calculations and reduce the amount of code needed, making our programs more efficient and concise. Here are some examples.
-
In finance, it’s used to discount future cash flows to their present value, which is crucial in evaluating the worth of investments.
-
In Computer Science, it can be used to calculate the complexity of algorithms and data structures, such as sorting and searching algorithms.
-
In kinetics, it helps describe the rate of chemical reactions using rate constants.
-
It models population growth in biology and the growth of microorganisms in biotechnology.
1. Python exponent operator: Double-Asterisk Method (x**n)
The double-asterisk method, also called the python exponent operator, is the most commonly used approach. It allows you to raise a number to a specific power with ease. This method is widely used due to its simplicity and intuitive nature.
You can use the python exponent operator to handle both integer and float values. Whether you need to calculate large numbers or perform complex mathematical operations, the double-asterisk method is efficient and performs exponentiation using arithmetic operators.
2. Built-In pow(x, n) Method
The pow(x, n) method in Python is a built-in function that calculates the exponentiation of a number. This is efficient and works using arithmetic operators. It offers additional flexibility by accepting a third argument for modular exponentiation.
One of the key advantages of the pow() function is its ability to handle integers, floats, and decimals. Whether you need to perform basic or advanced operations, the built-in pow() function is a suitable choice. It provides a convenient way without having to write complex code.
Additionally, it is worth mentioning that the pow() function can also handle negative numbers as exponents, allowing for greater versatility in mathematical calculations.
3. Python math exponent: math.pow(x, n) Method
The math.pow(x, n) method is a function from the math module in Python that calculates the exponentiation of a number. Similar to the built-in pow(x, n) function, this provides high precision and accuracy for exponent operations.
One key difference is that the math.pow() function requires importing the math module. This method is particularly useful when more advanced mathematical functions are required. It can handle both integer and float values as exponents, making it versatile for various types of calculations.
4. Python numpy exponent: numpy.power(x, n) Method
The numpy.power(x, n) method in the numpy library is specifically designed for operations on arrays or matrices. It efficiently calculates the exponentiation of an array or matrix, handling both integer and float values as exponents.
This is particularly useful in data science and scientific computing applications, where large datasets require efficient and optimized operations. With numpy.power(), you can easily perform the operation on arrays or matrices without the need for additional loops or logic.
5. Without exponential operator in Python or pow methods: Arithmetic multiplication Method
The arithmetic multiplication method is a fundamental operation in mathematics that involves multiplying two or more numbers together. While it may not be specifically designed for exponentiation like the math.pow() and numpy.power() methods, it can still be used to calculate exponents by repeatedly multiplying a number by itself.
This approach, known as repeated multiplication, works well for integer exponents but may result in loss of precision for floating-point exponents. Therefore, it is recommended to use the math.pow() or numpy.power() when precise exponentiation calculations are required. However, for simple cases where integer exponents are involved, you can use multiplication.
Comparison
As we saw, there are multiple ways to calculate exponents in Python. The double-asterisk method, built-in pow(), math.pow(), and numpy.power() are commonly used. The choice of exponentiation method depends on factors such as performance, precision, and compatibility with other libraries.
-
Numpy.power() is specifically designed for performing exponentiation operations on arrays or matrices
-
Math.pow() provides high precision and accuracy.
-
The double-asterisk method is the simplest and most intuitive.
-
Use repeated multiplication for custom algorithms or very large exponents, where computational efficiency or memory usage is a concern. Understanding these differences ensures efficient and accurate exponentiation calculations.
Which python exponent method is the most efficient?
The efficiency of an exponentiation method depends on the specific use case and input values. The double-asterisk method (x**n) is generally considered the most efficient for basic exponentiation. However, for complex mathematical functions and precision requirements, math.pow() or numpy.power() may be preferred. It’s important to benchmark and compare methods for specific scenarios to determine the most efficient one.
Common Errors While Calculating Exponents in Python
One common error is the omission of the double-asterisk operator (**), which is used for exponentiation.
Another mistake is mistyping the exponent or using incorrect syntax. It is crucial to use the correct order of operations to ensure accurate results when calculating exponents. Mixing integer and float values as operands can lead to unexpected behavior or loss of precision.
Additionally, importing the required modules, such as math or numpy, incorrectly can cause errors. Avoiding these errors will help ensure the accuracy and reliability of exponent calculations.
How to avoid these errors?
To avoid errors when calculating exponents in Python, utilize the double-asterisk exponent operator for efficient exponentiation. Take advantage of Python’s math module and its pow function. Be cautious with large values to prevent overflow errors. Additionally, consider using libraries like NumPy for more advanced exponentiation operations. Ensure that input values are within Python’s range of handling.
Advanced Exponentiation Techniques
Advanced exponentiation techniques are more efficient and optimized methods. These techniques are particularly useful when dealing with large exponents or very large numbers. Some advanced techniques include:
-
Squaring Technique: This technique reduces the number of multiplications required by leveraging the binary representation of the exponent. It’s commonly used and is also implemented in Python’s built-in ** operator and pow() function.
-
Fast Modular Technique: Important in cryptography and number theory, it efficiently computes exponentiation modulo another number, reducing memory and computation requirements.
-
Binary Technique: A variation of squaring technique, it’s useful for non-integer exponents and breaks down the exponent into binary digits for efficient calculation.
-
Matrix Technique: Used for exponentiating matrices, this technique finds applications in computer graphics and optimization problems.
Exponents with complex numbers
Yes, exponentiation with complex numbers is supported. You can use the pow function to perform exponentiation with complex numbers. Understanding the rules and properties of imaginary units is important when exponentiating complex numbers. Experimenting with complex number arithmetic and exponentiation in Python will help you explore their unique characteristics compared to real number exponentiation.
Conclusion
In conclusion, understanding exponents is crucial in programming as it allows you to perform calculations with large or small numbers efficiently. Python provides different ways to calculate them, including the double-asterisk method, built-in pow(), math.pow(), and numpy.power(). Each method has its advantages and efficiency, so it’s important to choose the right one for your specific needs. Additionally, be aware of common errors that can occur while calculating exponents in Python and learn how to avoid them. Lastly, if you’re looking to explore advanced exponentiation techniques, you can even use exponents with complex numbers. Keep exploring and experimenting with python exponents to enhance your programming skills.