· 7 min read
Rust
Discover Rust: Safety, performance, concurrency in modern systems language. Memory safety, parallel support, versatile syntax. Explore use cases.
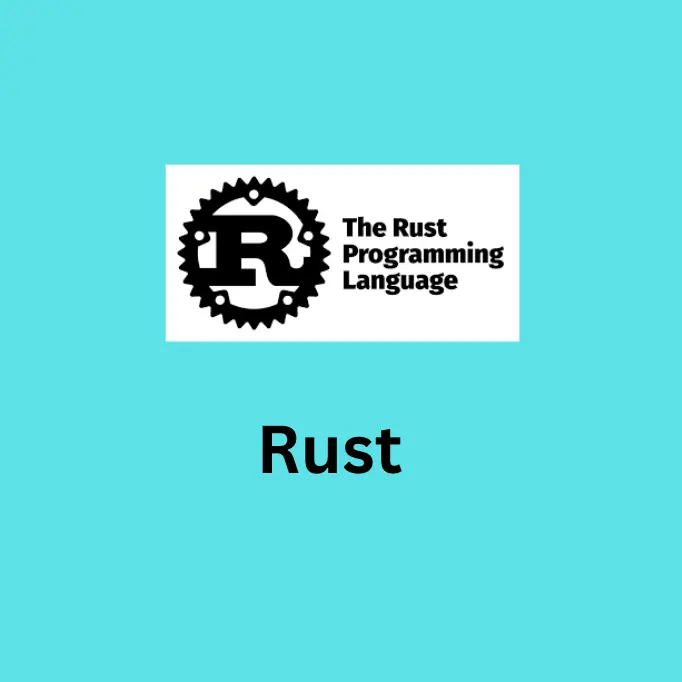
Rust programming language started in the year 2010, it was initially designed and developed by Graydon Hoare, a Mozilla employee. The first official version, Rust 0.1, was released on January 5th, 2012. The language has been actively developed since then, with new versions and updates released regularly. Rust was originally developed as an experiment in creating a new system programming language that would address some of the problems with existing languages such as C and C++.
Advantages of Rust
Rust has several advantages, including:
-
Memory safety: Rust has a strong focus on preventing common programming errors such as null or dangling pointer references, which can lead to memory safety issues. It provides a borrow checker that ensures that references to data will always be valid.
-
Performance: Rust is a compiled language and has a low-level control over the system, which allows for efficient and fast code execution.
-
Concurrency: Rust provides a number of features that make it easy to write concurrent and parallel code. It has built-in support for lightweight “green” threads, and its ownership model makes it easy to write concurrent code without the need for locks or other synchronization mechanisms.
-
Cross-platform compatibility: Rust can be used to develop applications for a wide range of platforms, including Windows, macOS, and Linux, as well as for embedded systems and web assembly.
-
Community and ecosystem: Rust has an active and growing community of developers who contribute to the language and its ecosystem, which provides a wide range of libraries and tools for common tasks.
-
Statically typed: Rust is a statically typed language which means that it can catch errors at compile-time, which makes it easier to develop and maintain large projects.
-
Efficient and low-level control: Rust provides low-level control over the system, which allows for efficient and fast code execution, and also provides a way to interact with C code, making it possible to use existing C libraries in your Rust projects.
Rust vs C
Rust and C are both systems programming languages, but they have some key differences:
-
Memory safety: Rust has a strong focus on preventing common programming errors such as null or dangling pointer references, which can lead to memory safety issues. It provides a borrow checker that ensures that references to data will always be valid. C, on the other hand, does not have this level of built-in safety and requires manual memory management, which can make it more prone to errors.
-
Concurrency: Rust has built-in support for lightweight “green” threads, and its ownership model makes it easy to write concurrent code without the need for locks or other synchronization mechanisms. C does not have this level of built-in support and requires manual synchronization, which can be more complex to implement.
-
Syntax: Rust has a more modern and expressive syntax than C, which can make it easier to write and read code. C has a more traditional and low-level syntax, which can make it more difficult to understand.
-
Type safety: Rust is a statically typed language which means that it can catch errors at compile-time, which makes it easier to develop and maintain large projects. C is less restrictive and allows for more flexibility, but this also means that it can be more prone to errors.
-
Interoperability: C has been around for decades and has a large number of libraries and frameworks available, making it easy to find existing code to use in your projects. Rust is newer and has a smaller ecosystem but it also provides a way to interact with C code, making it possible to use existing C libraries in your Rust projects.
-
Performance: Both Rust and C are compiled languages and have the ability to produce highly efficient machine code, but Rust has a more modern and expressive syntax, which allows for more efficient high-level abstractions.
Both languages have their own strengths and weaknesses, and the choice between Rust and C often depends on the specific requirements of the project and the preferences of the developer.
Use cases for Rust
Rust is best suited for use cases that require:
-
Memory safety: Rust has a strong focus on preventing common programming errors such as null or dangling pointer references, which can lead to memory safety issues. It provides a borrow checker that ensures that references to data will always be valid. This makes it a great choice for systems programming and other performance-critical applications where memory safety is a concern.
-
Concurrency and parallelism: Rust has built-in support for lightweight “green” threads, and its ownership model makes it easy to write concurrent code without the need for locks or other synchronization mechanisms. This makes it a great choice for applications that need to take full advantage of multi-core processors.
-
Performance: Rust is a compiled language and has a low-level control over the system, which allows for efficient and fast code execution. This makes it a great choice for systems programming and other performance-critical applications.
-
Cross-platform compatibility: Rust can be used to develop applications for a wide range of platforms, including Windows, macOS, and Linux, as well as for embedded systems and web assembly.
-
Systems programming: Rust is designed to be efficient and low-level, which makes it a great choice for systems programming tasks such as writing operating systems, device drivers, and embedded systems.
-
Networking: Rust’s focus on safety and concurrency makes it a great choice for networking applications, such as servers, proxies, and routers.
-
Game development: Rust has been used in the game industry for various tasks, from game engines to game logic, Rust’s performance and memory safety make it a great choice for game development.
-
Blockchain and Cryptography: Rust has a growing ecosystem of libraries for blockchain and cryptography and its safety features make it a great choice for building secure and reliable blockchain and crypto-related applications.
It’s worth noting that Rust’s growing ecosystem and its community are making it more and more suitable for various use cases, and it’s being adopted by many organizations in various industries.
When not to use the Rust programming language
Rust is a versatile systems programming language and can be used for a wide range of applications, but there are some use cases where it may not be the best choice:
-
High-performance numerical computing: Rust has good performance characteristics, but there are other languages such as C++, FORTRAN, and Julia that are specifically designed for high-performance numerical computing and may be a better choice for this type of work.
-
Embedded systems with limited resources: Rust is designed to be efficient, but it has a larger runtime and memory footprint than languages like C or Assembly, which may be a better choice for embedded systems with limited resources.
-
Rapid prototyping or scripting: Rust is a compiled language and has a relatively large setup and build time which may not be suitable for rapid prototyping or scripting.
-
Server-side web development: Rust can be used for web development, but it has a relatively small ecosystem of web development libraries and frameworks compared to more established languages like JavaScript, Python, and Ruby.
-
GUI development: Rust has a relatively small ecosystem of GUI libraries and frameworks compared to other languages like C++, C#, and Java.
However, as the Rust ecosystem and its community are growing, it’s getting more and more suitable for various use cases.
It’s worth noting that Rust’s memory safety and performance characteristics make it a great choice for systems programming, networking, embedded systems, and other performance-critical applications.
Sample Code
Rust function to determine if a number is prime.
fn is_prime(n: i32) -> bool { if n <= 1 { return false; } for i in 2..(n as f32).sqrt() as i32 + 1 { if n % i == 0 { return false; } } true }
This function takes in an argument of type
and returns a “. It checks if the input number is prime by dividing it from 2 to the square root of the number and if it finds any divisor other than 1 and itself it returns false, otherwise it returns true.
“